Easy Screen Shake that Works With Any Renderer (Example Link Included)
PROGRAMMINGGAME DEVELOPMENTGAME DESIGN
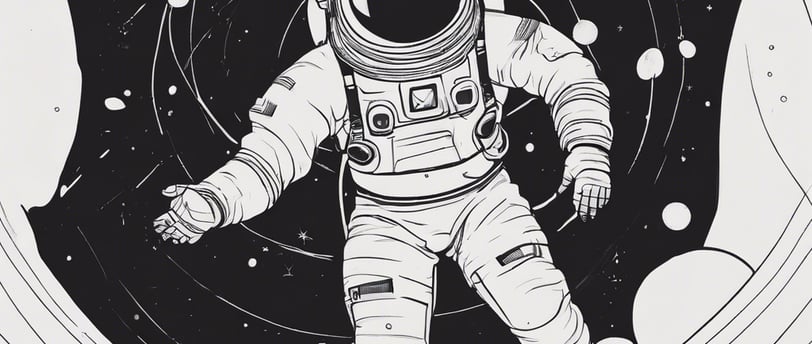
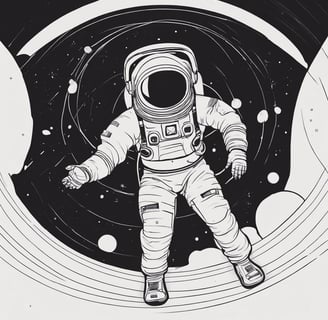
The current game project, Frontline Fantasy, is missing much of the pizzazz that comes out of the box when using a Game Engine (Read this post on why I am not using one). I started analyzing other games and discovered one feature that could add some juice to the playing experience is a well-timed screen shake. When I searched Google for a general implementation, only unity or complicated examples popped up. Screen shake shouldn't be that hard to implement.
The solution I came up with is not only simple, but it can also be implemented with any graphics library.
Pseudocode
See GitHub Repo for implementation using Go+SDL2
Pseudocode Breakdown
This code is just a general outline of how I got screen shake to work. It will not compile (as far as I know) as any programming language. I am going to explain each part so that anyone can implement it in any language and for any graphics library/renderer, but if you would like an example using Go+SDL, go to this section.
The Screen Type
The Screen type is used as a general container for all other game entities. Though I use the word entity here, this does not require an entity/component system. An entity is just a graphic object with a position on the screen. The entities' position for display is based on their parent Screen. The Screen X and Y should be Zero, Zero outside of shaking.
The Shake Function
The Shake function initializes the screen shake. It sets the variables needed to run the shake and sets Shaking to true. In most games, update statements are run every frame so the Shaking variable is used to control when updateShake should be calculated.
The parameter duration determines how long the shake should occur. For me, 300-500 milliseconds was a good duration.
The intensity parameter determines how far the screen will offset each shake. 1-3 pixels was a good amount for what I needed.
The last variable is shakerSwitch which is how I determine the direction of the X, Y offset. This is used to shake the screen back and forth.
The updateShake Function
This function is included in every update call for the game. Since this will not need to run every frame, the `Shaking` variable will be used to exclude it when not necessary. The deltaTime is a common parameter used in update statements for games. Usually, this is used to normalize things according to framerate (which should also be done for the actual implementation), but for this instance, it is used to shake until the shakeDuration is reached.
After the `Shaking` value is checked, the shakeCounter is checked to determine if the shaking is complete. shakeCounter is updated by adding deltaTime. If the duration is reached, then the variables are reset.
Next, the Screen is updated by offsetting the X and Y properties by the intensity. The offset is flipped back and forth by the shakerSwitch variable which is updated by multiplying by -1 only when the screen X and Y are set back to zero.
That's All That is Needed
Screen shake does not have to be complicated. All it is is changing the position of a container and its children, back and forth, really fast. The top examples I found were all related to Unity. When searching specifically for SDL2 screen shake, the examples provided were dealing directly with how a texture is rendered and still really specific to SDL2. With this general approach, I can implement this in anything and not be tied to a library.
Good Instances to use Screen Shake
Screen shake is a powerful tool when developing a game. It can make actions and effects feel more impactful. Common uses are for explosions, dealing or taking damage, and environmental events.
For Frontline Fantasy, fishing with dynamite feels a lot better when the dynamite hits, and the screen shakes.
Example GitHub Repository
This implementation uses Go+SDL2 to demonstrate how the pseudocode could be used:
Edits:
Thanks u/partybusiness for showing me bug in code